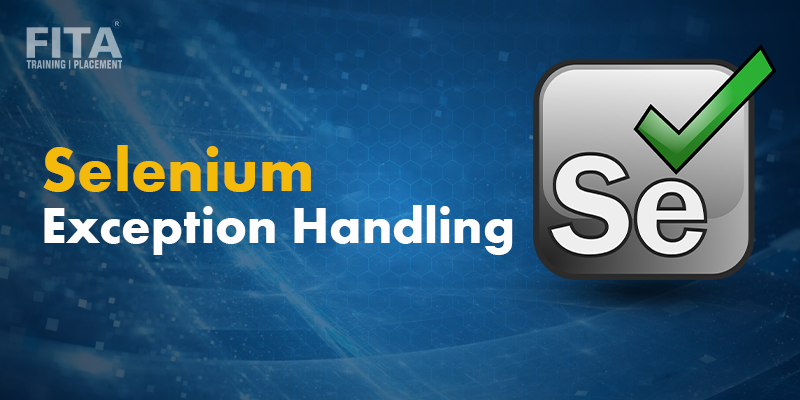
Selenium WebDriver has come to be a critical tool for automating net browser interactions. It empowers testers and developers to streamline the testing process, enables to identify regressions quickly, and build solid web applications. But even the most meticulously crafted Selenium scripts can come upon roadblocks – exceptions. If not handled gracefully, these unexpected errors can bring your automation journey to a screeching halt.
This blog delves deep into the case studies of Selenium exceptions, equipping you with the knowledge and strategies to overcome these challenges with confidence. We'll explore the the one-of-a-kind types of webdriver exception in Selenium, exception handling in Selenium, and strategies to make your scripts resilient and informative.
Why Exception Handling Matters in Selenium
Imagine this scenario: your Selenium script is working its way through a test case, clicking buttons, and entering text. Suddenly, it throws an exception in Selenium. The test abruptly stops, leaving you with a cryptic error message and a sense of frustration. Without proper handling of webdriver exception in selenium, your entire test suite might crumble like a house of cards at the first hurdle.
Here's why mastering exception handling in Selenium is crucial:
1. Ensures Test Stability
Selenium exceptions can arise for various reasons, such as network issues, page load delays, or unexpected changes in the web application's UI. By implementing robust exception handling in Selenium, you prevent these errors from derailing your entire test suite. Even if one test encounters an issue, the others can continue execution, providing valuable insights.
2. Improves Debugging Efficiency
Exception handling in Selenium provides a safety net. When an error occurs, informative messages are displayed, pinpointing the exact line of code where the issue arose. This streamlines the debugging process, saving you time and effort in pinpointing the root cause.
3. Enhances Test Reporting
Exception handling in Selenium allows you to capture and log error messages. This enriched information can be integrated into your test reports, providing a clearer picture of the test execution. This is particularly beneficial for collaborative testing environments and identifying recurring issues.
Common Selenium Exceptions
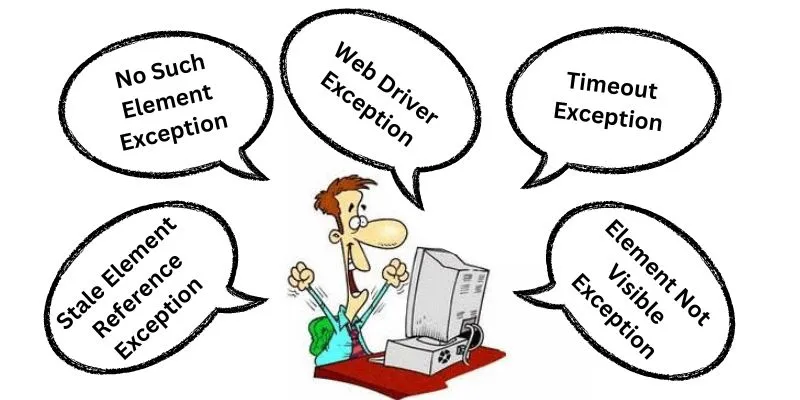
Selenium throws a variety of exceptions, each with a specific meaning. Here's a breakdown of some of the most frequently encountered Selenium exceptions:
1. No Such Element Exception
This exception occurs when your script attempts to interact with an element that doesn't exist on the webpage. Common reasons include typos in your locators, elements being dynamically loaded after the page has initially rendered, or the element being removed from the DOM entirely.
2. Stale Element Reference Exception
This exception arises when the script tries to interact with an element that has become stale – meaning, the element's properties (like ID or attributes) have changed since it was first located. This can happen due to page refreshes or dynamic content updates.
3. Web Driver Exception
This is a broad category encompassing various errors related to the webdriver exception in selenium itself, such as issues initialising the WebDriver instance, driver not found exceptions, or problems interacting with the browser.
4. Timeout Exception
This exception signifies that an expected condition (like an element becoming visible) didn't occur within the specified timeframe. This can be due to slow network connections, overloaded servers, or complex web page elements that take longer to load.
5. Element Not Visible Exception
This exception gets thrown when your script attempts to interact with an element that is present on the page but is hidden from view. This could be due to the element being located behind another element or having its visibility style set to "hidden."
Understanding the nature of these Selenium exceptions empowers you to implement targeted exception handling in Selenium. To master selenium, try pursuing Selenium Training in Chennai.
Techniques for Exception Handling in Selenium
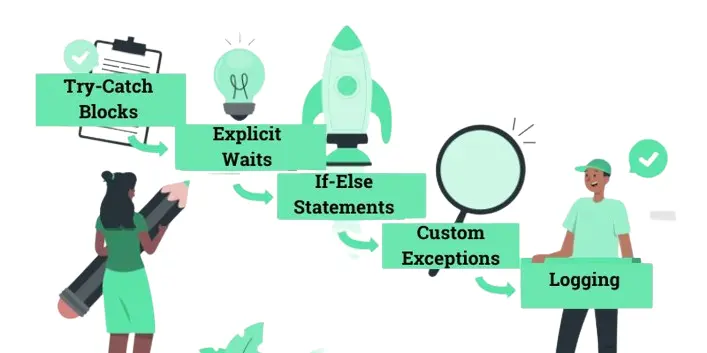
Now that we've identified the familiar foes let's explore the weapons in your arsenal for exception handling in Selenium:
1. Try-Catch Blocks
This is the bread and butter of exception handling in Selenium. You wrap the code susceptible to exceptions within a `try` block. If any exception occurs, the corresponding `catch` block is executed, allowing you to take appropriate actions like logging the error, retrying the operation, or gracefully failing the test.
try {
// Code that might throw an exception
driver.findElement(By.id("nonexistent_element")).click();
} catch (NoSuchElementException e) {
System.out.println("Element with ID 'nonexistent_element' not found!");
}
2. Explicit Waits
Selenium exceptions often arise because your script tries to interact with elements before they're fully loaded or become visible. Explicit waits provide a solution. You specify a condition (like element visibility) and a timeout. The WebDriver waits until the condition is met before proceeding, preventing webdriver exception in selenium.
WebDriverWait wait = new WebDriverWait(driver, 10);
WebElement element = wait.until(ExpectedConditions.visibilityOfElementLocated(By.id("myElement")));
element.click();
3. If-Else Statements
webdriver exception in selenium can also be handled using if-else statements. You can check if an element exists before attempting to interact with it. This approach can be simpler for basic scenarios but might become cumbersome for complex test cases.
if (driver.findElements(By.id("myElement")).size() > 0) {
driver.findElement(By.id("myElement")).click();
} else {
System.out.println("Element with ID 'myElement' not found!");
}
4. Custom Exceptions
For specific scenarios, you might want to create custom exceptions. This allows you to define your own error messages and handle them in a centralized manner within your test framework.
public class MyCustomException extends Exception {
public MyCustomException(String message) {
super(message);
}
}
// In your test case
try {
// Code that might throw a custom exception
if (! someCondition) {
throw new MyCustomException("Custom exception message!");
}
} catch (MyCustomException e) {
System.out.println(e.getMessage());
}
5. Logging
Exception handling in Selenium should go hand-in-hand with robust logging practices. When an exception occurs, log the error message, stack trace, and any relevant information about the test case. This facilitates debugging and provides a historical record of test execution issues.
try {
// Code that might throw an exception
driver.findElement(By.name("submit_button")).click();
} catch (Exception e) {
logger.error("Error occurred while clicking submit button!", e);
}
These techniques are better when you ideally learn from an industry expert trainer to be handy when doubts occur. Our Selenium Training in Madurai makes this possible, bringing leading working professionals into the industry.
Advanced Exception Handling Strategies
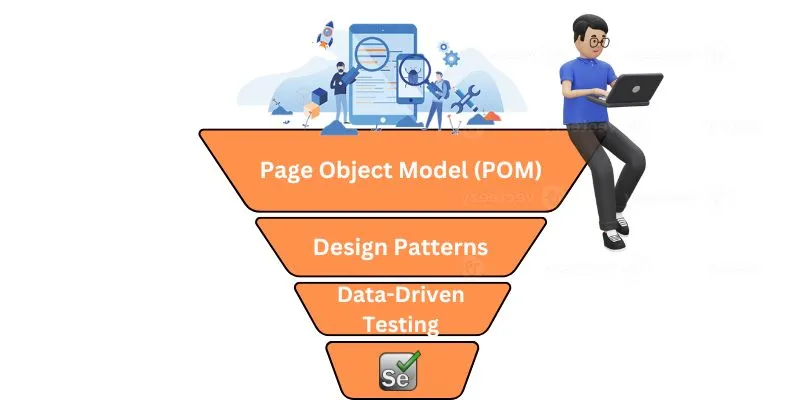
As your test automation endeavours mature, consider these advanced exception handling in Selenium techniques:
- Page Object Model (POM)
By encapsulating UI elements and interactions within reusable classes, POM promotes better code organisation and reduces the likelihood of webdriver exception in selenium.
- Design Patterns
Implementing design patterns like the Retry pattern allows you to automatically retry failed operations several times before marking the test as failed.
- Data-Driven Testing
Separating test data from your test scripts makes your tests more maintainable and reduces the risk of exceptions in Selenium caused by invalid data.
To learn more about advanced exception handling in Selenium, pursue our Selenium Training in Coimbatore.
Specific Exception Handling Scenarios
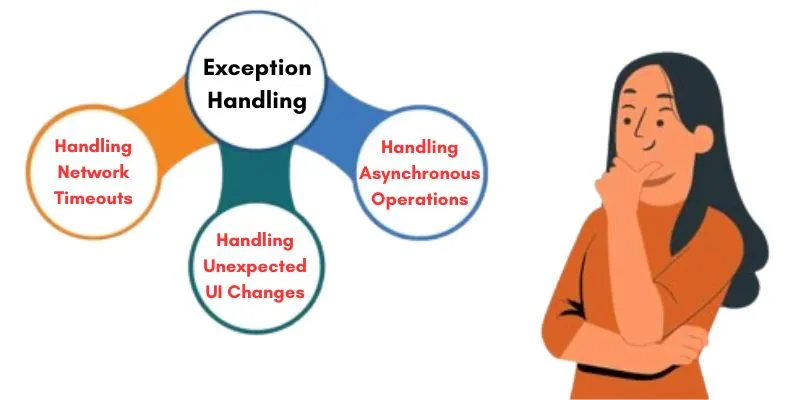
Let's explore some common scenarios and how to handle them effectively using exception handling in Selenium:
1. Handling Network Timeouts
Network issues can lead to TimeoutException during test execution. Here's how to address them:
- Increase Implicit Wait
Selenium provides an implicit wait that applies to all findElement and findElements commands. Setting a higher implicit wait time allows the WebDriver to wait for elements to load before throwing a TimeoutException. However, this approach can impact overall test execution speed.
- Implement Explicit Waits with WebDriverWait
As discussed earlier, explicit waits offer more granular control. You can configure a WebDriverWait with a specific timeout for a particular condition, like waiting for an element to be clickable before proceeding.
- Custom Exception Handling
For scenarios where network timeouts are expected (e.g., testing slow-loading pages), create a custom exception that inherits from TimeoutException. This allows you to differentiate network-related timeouts from other waiting issues and handle them in a specific manner within your test framework.
2. Handling Unexpected UI Changes
Web applications are subject to change. Here's how to make your scripts adaptable:
- Use Relative Locators
Webdriver exception in selenium often arise due to changes in element identifiers. Employing relative locators based on element attributes or relationships with other elements can enhance script resilience.
- Wait for Element Presence Before Interaction
NoSuchElementException can occur if elements are dynamically added to the page. Implement explicit waits that check for the element's existence before attempting to interact with it.
- Implement Page Factory Pattern
The Page Factory pattern from Selenium WebDriver helps manage UI elements and locators in a centralized manner. This facilitates easier maintenance and reduces the impact of UI changes on your scripts.
3. Handling Asynchronous Operations
Modern web applications heavily rely on asynchronous operations (AJAX, etc.) that can lead to StaleElementReferenceException. Here's how to tackle them:
- Use JavaScriptExecutor
Selenium provides the JavaScriptExecutor interface to execute JavaScript code within the browser. You can use this to interact with elements that haven't fully loaded in the DOM yet, preventing StaleElementReferenceException.
- Wait for Asynchronous Operations to Complete
Libraries like Selenium WebDriverWait offer utilities like `ExpectedConditions.presenceOfAllElementsLocated` that wait until all elements matching a locator are present and visible, ensuring asynchronous operations have finished before script execution proceeds.
- Utilise Asynchronous Testing Frameworks
Frameworks like Selenium WebDriver with WebDriverWait offer asynchronous testing capabilities. These frameworks handle asynchronous operations automatically, simplifying test creation and reducing the risk of StaleElementReferenceException.
Enrolling in Selenium Training in Bangalore is always recommended to learn hands-on exception selenium handling.
Leveraging Frameworks and Tools for Enhanced Exception Handling
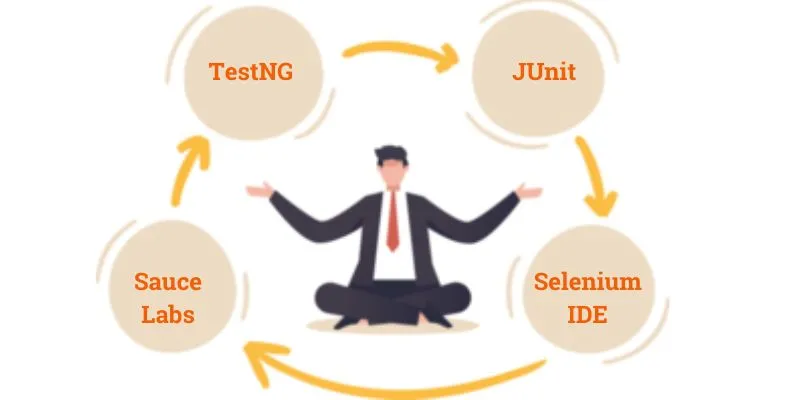
Several frameworks and tools can elevate your exception handling in Selenium practices:
- TestNG
This popular testing framework provides built-in support for exception handling. You can use annotations like `@ExpectedException` to specify the expected exception type and verify if it occurs during test execution.
- JUnit
While not as robust as TestNG for exception handling, JUnit allows you to use try-catch blocks within your test methods. Additionally, libraries like ExpectedConditions can be integrated with JUnit for more advanced waiting strategies.
- Selenium IDE
This browser extension allows you to record and playback Selenium scripts. It offers basic exception handling capabilities like logging errors and continuing test execution after encountering exceptions.
- Sauce Labs
Cloud-based testing platforms like Sauce Labs provide detailed error logs and screenshots whenever exceptions in Selenium occur. This facilitates remote debugging and troubleshooting across different browsers and operating systems.
By leveraging frameworks and tools alongside continuous refinement of your approach, you can streamline webdriver exception in selenium and gain valuable insights into test failures. Selenium Training in Salem can equip you with the knowledge and best practices to tackle these challenges. As your test suite and web applications evolve, so should your exception handling strategies. With the knowledge provided here, you can conquer Selenium exceptions and build robust test automation. Happy automating!